In case of Debian Squeeze,
after going into the install steps, proceed until loading the device drivers.
Then, if pushed Ctrl+Alt+F2, you can get the command prompt.
# fdisk -l
# mount -t ext4 /dev/XXX /chroot
# mount --bind /dev /chroot/dev
# mount -t sysfs none /chroot/sys
# mount -t proc none /chroot/proc
# chroot /chroot/
# grub-install /dev/XXX
Welcome
Wheresoever you go, go with all your heart. (Confucius)
10/11/2011
Single instance in MFC
Sometimes you want to make your application only with a single instance.
Unfortunately MFC don't provide any APIs. However, there is the simplest method by MUTEX.
First, you create the globally unique identifier (GUID) to create MUTEX.
MFC provides creating GUID: Tools-Create GUID.
after creating it, just copy and paste in your code.
The following code located in InitInstance() of MFC.
Unfortunately MFC don't provide any APIs. However, there is the simplest method by MUTEX.
First, you create the globally unique identifier (GUID) to create MUTEX.
MFC provides creating GUID: Tools-Create GUID.
after creating it, just copy and paste in your code.
The following code located in InitInstance() of MFC.
CString stringGUID = L"........";
HANDLE hMutex = CreateMutex(NULL, TRUE, stringGUID);
if( GetLastError() == ERROR_ALREADY_EXISTS )
{
CloseHandle(hMutex);
return FALSE;
}
6/03/2011
libconfig - C/C++ Configuration File Library
Libconfig is a great library which can process configuration files base on structured format such as XML.
According tohttp://www.hyperrealm.com/libconfig/, this file format is more compact and more readable than XML. Advantages are the follows:
I tested whether or not it works on Windows 7 and Visual studio 2005. The result is very successful.
Here is a sample configuration file.
As shown in the sample configuration above, this is so very powerful that I love the libconfig library.
Finally, I provide a sample code to read a configuration file.
According tohttp://www.hyperrealm.com/libconfig/, this file format is more compact and more readable than XML. Advantages are the follows:
- type-aware: by parser, it is automatically bound to the appropriate type such as int, float, array, and string.
- support both the C and C++
- works on various operating systems: GNU/Linux, Mac OS X, Solaris, FreeBSD, and Windows (2000, XP, and later)
I tested whether or not it works on Windows 7 and Visual studio 2005. The result is very successful.
Here is a sample configuration file.
#---------------------------- # Example Configuration File #--------------------------- # application: { /* This section defines some settings for our * main application window, such as size and * position. */ window: { title = "My Application"; size = { /* width */ w = 640; /* height */ h = 480; }; pos = { x = 350; y = 250; }; }; a = 5; b = 6; ff = 1E6; test-comment = "/* hello\n \"there\"*/"; test-long-string = "A very long string that spans multiple lines. " /* but wait, there's more... */ "Adjacent strings are automatically" " concatenated."; test-escaped-string = "\"This is\n a test.\""; group1: { x = 5; y = 10; my_array = [ 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21, 22 ]; flag = TRUE; group2: { zzz = "this is a test"; }; states = [ "CT", // Connecticut "CA", // California "TX", // Texas "NV", // Nevada "FL" // Florida ]; }; /* this would cause an error */ // a = "hi!"; }; binary = [ 0xAA, 0xBB, 0xCC ]; list = ( ( "abc", 123, true ), 1.234, ( /* an empty list */ ) ,[ 1, 2, 3 ], { a = (1, 2, true); } ); books = ( "inventory", { title = "Treasure Island"; author = "Robert Louis Stevenson"; price = 29.99; qty = 5; }, { title = "Snow Crash"; author = "Neal Stephenson"; price = 9.99; qty = 8; }, { } ); # miscellaneous stuff misc: { port = 5000; pi = 3.14159265; enabled = FALSE; mask = 0xAABBCCDD; unicode = "STARGΛ̊TE SG-1"; // UTF-8 string bigint = 9223372036854775807L; bighex = 0x1122334455667788L; }; ### eof
As shown in the sample configuration above, this is so very powerful that I love the libconfig library.
Finally, I provide a sample code to read a configuration file.
#include <iostream>#include <iomanip>#include <cstdlib>#include <libconfig.h++>using namespace std;using namespace libconfig;// This example reads the configuration file 'example.cfg' and displays// some of its contents.int main(int argc, char **argv){Config cfg;// Read the file. If there is an error, report it and exit.try{cfg.readFile("example.cfg");}catch(const FileIOException &fioex){std::cerr << "I/O error while reading file." << std::endl;return(EXIT_FAILURE);}catch(const ParseException &pex){std::cerr << "Parse error at " << pex.getFile() << ":" << pex.getLine()<< " - " << pex.getError() << std::endl;return(EXIT_FAILURE);}// Get the store name.try{string name = cfg.lookup("name");cout << "Store name: " << name << endl << endl;}catch(const SettingNotFoundException &nfex){cerr << "No 'name' setting in configuration file." << endl;}const Setting& root = cfg.getRoot();// Output a list of all books in the inventory.try{const Setting &books = root["inventory"]["books"];int count = books.getLength();cout << setw(30) << left << "TITLE" << " "<< setw(30) << left << "AUTHOR" << " "<< setw(6) << left << "PRICE" << " "<< "QTY"<< endl;for(int i = 0; i < count; ++i){const Setting &book = books[i];// Only output the record if all of the expected fields are present.string title, author;double price;int qty;if(!(book.lookupValue("title", title)&& book.lookupValue("author", author)&& book.lookupValue("price", price)&& book.lookupValue("qty", qty)))continue;cout << setw(30) << left << title << " "<< setw(30) << left << author << " "<< '$' << setw(6) << right << price << " "<< qty<< endl;}cout << endl;}catch(const SettingNotFoundException &nfex){// Ignore.}// Output a list of all books in the inventory.try{const Setting &movies = root["inventory"]["movies"];int count = movies.getLength();cout << setw(30) << left << "TITLE" << " "<< setw(10) << left << "MEDIA" << " "<< setw(6) << left << "PRICE" << " "<< "QTY"<< endl;for(int i = 0; i < count; ++i){const Setting &movie = movies[i];// Only output the record if all of the expected fields are present.string title, media;double price;int qty;if(!(movie.lookupValue("title", title)&& movie.lookupValue("media", media)&& movie.lookupValue("price", price)&& movie.lookupValue("qty", qty)))continue;cout << setw(30) << left << title << " "<< setw(10) << left << media << " "<< '$' << setw(6) << right << price << " "<< qty<< endl;}cout << endl;}catch(const SettingNotFoundException &nfex){// Ignore.}return(EXIT_SUCCESS);}
libconfig - C/C++ Configuration File Library
Libconfig is a great library which can process configuration files base on structured format such as XML.
According tohttp://www.hyperrealm.com/libconfig/, this file format is more compact and more readable than XML. Advantages are the follows:
I tested whether or not it works on Windows 7 and Visual studio 2005. The result is very successful.
Here is a sample configuration file.
As shown in the sample configuration above, this is so very powerful that I love the libconfig library.
Finally, I provide a sample code to read a configuration file.
According tohttp://www.hyperrealm.com/libconfig/, this file format is more compact and more readable than XML. Advantages are the follows:
- type-aware: by parser, it is automatically bound to the appropriate type such as int, float, array, and string.
- support both the C and C++
- works on various operating systems: GNU/Linux, Mac OS X, Solaris, FreeBSD, and Windows (2000, XP, and later)
I tested whether or not it works on Windows 7 and Visual studio 2005. The result is very successful.
Here is a sample configuration file.
#---------------------------- # Example Configuration File #--------------------------- # application: { /* This section defines some settings for our * main application window, such as size and * position. */ window: { title = "My Application"; size = { /* width */ w = 640; /* height */ h = 480; }; pos = { x = 350; y = 250; }; }; a = 5; b = 6; ff = 1E6; test-comment = "/* hello\n \"there\"*/"; test-long-string = "A very long string that spans multiple lines. " /* but wait, there's more... */ "Adjacent strings are automatically" " concatenated."; test-escaped-string = "\"This is\n a test.\""; group1: { x = 5; y = 10; my_array = [ 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21, 22 ]; flag = TRUE; group2: { zzz = "this is a test"; }; states = [ "CT", // Connecticut "CA", // California "TX", // Texas "NV", // Nevada "FL" // Florida ]; }; /* this would cause an error */ // a = "hi!"; }; binary = [ 0xAA, 0xBB, 0xCC ]; list = ( ( "abc", 123, true ), 1.234, ( /* an empty list */ ) ,[ 1, 2, 3 ], { a = (1, 2, true); } ); books = ( "inventory", { title = "Treasure Island"; author = "Robert Louis Stevenson"; price = 29.99; qty = 5; }, { title = "Snow Crash"; author = "Neal Stephenson"; price = 9.99; qty = 8; }, { } ); # miscellaneous stuff misc: { port = 5000; pi = 3.14159265; enabled = FALSE; mask = 0xAABBCCDD; unicode = "STARGΛ̊TE SG-1"; // UTF-8 string bigint = 9223372036854775807L; bighex = 0x1122334455667788L; }; ### eof
As shown in the sample configuration above, this is so very powerful that I love the libconfig library.
Finally, I provide a sample code to read a configuration file.
#include <iostream>#include <iomanip>#include <cstdlib>#include <libconfig.h++>using namespace std;using namespace libconfig;// This example reads the configuration file 'example.cfg' and displays// some of its contents.int main(int argc, char **argv){Config cfg;// Read the file. If there is an error, report it and exit.try{cfg.readFile("example.cfg");}catch(const FileIOException &fioex){std::cerr << "I/O error while reading file." << std::endl;return(EXIT_FAILURE);}catch(const ParseException &pex){std::cerr << "Parse error at " << pex.getFile() << ":" << pex.getLine()<< " - " << pex.getError() << std::endl;return(EXIT_FAILURE);}// Get the store name.try{string name = cfg.lookup("name");cout << "Store name: " << name << endl << endl;}catch(const SettingNotFoundException &nfex){cerr << "No 'name' setting in configuration file." << endl;}const Setting& root = cfg.getRoot();// Output a list of all books in the inventory.try{const Setting &books = root["inventory"]["books"];int count = books.getLength();cout << setw(30) << left << "TITLE" << " "<< setw(30) << left << "AUTHOR" << " "<< setw(6) << left << "PRICE" << " "<< "QTY"<< endl;for(int i = 0; i < count; ++i){const Setting &book = books[i];// Only output the record if all of the expected fields are present.string title, author;double price;int qty;if(!(book.lookupValue("title", title)&& book.lookupValue("author", author)&& book.lookupValue("price", price)&& book.lookupValue("qty", qty)))continue;cout << setw(30) << left << title << " "<< setw(30) << left << author << " "<< '$' << setw(6) << right << price << " "<< qty<< endl;}cout << endl;}catch(const SettingNotFoundException &nfex){// Ignore.}// Output a list of all books in the inventory.try{const Setting &movies = root["inventory"]["movies"];int count = movies.getLength();cout << setw(30) << left << "TITLE" << " "<< setw(10) << left << "MEDIA" << " "<< setw(6) << left << "PRICE" << " "<< "QTY"<< endl;for(int i = 0; i < count; ++i){const Setting &movie = movies[i];// Only output the record if all of the expected fields are present.string title, media;double price;int qty;if(!(movie.lookupValue("title", title)&& movie.lookupValue("media", media)&& movie.lookupValue("price", price)&& movie.lookupValue("qty", qty)))continue;cout << setw(30) << left << title << " "<< setw(10) << left << media << " "<< '$' << setw(6) << right << price << " "<< qty<< endl;}cout << endl;}catch(const SettingNotFoundException &nfex){// Ignore.}return(EXIT_SUCCESS);}
6/02/2011
Windows 8: Building Video
Microsoft revealed a new operating system, windows 8.
The below movie shows the working by Touch Screen.
Maybe, it is optimized for Tablet PC.
The below movie shows the working by Touch Screen.
Maybe, it is optimized for Tablet PC.
6/01/2011
Automatically Scheduling of SyncToy
SyncToy doesn't have the feature to allow user to schedule folders to run synchronization process.
But, we can use Task Scheduler of Windows 7.
[Procedure]
But, we can use Task Scheduler of Windows 7.
[Procedure]
- Click Task Scheduler, Location: Start menu - All Programs - Accessories - System Tools - Task Scheduler
- Click Create Basic Task in the Actions
- Type in a Name and Description
- Click Next button
- Select the frequency of when you want the task to start
- Click Next button
- Input date and times
- Click Next button
- Select Start a program
- Click Next button
- In Program/script, Locate the SyncToyCmd.exe
- In Add arguments, Type -R which run all folder pairs that are active for run all. If you want to run just a single folder pair, add -R"Folder pair name" to the end of the command line
- Click Next button
log4cplus - Logging library to C++ such as log4j
log4cplus is the excellent logging library to C++ such as log4j.
You can see at log4cplus.sourceforge.net.
Current version is 1.0.4, released on January 2011.
I tested this library on Visual studio 2005.
It is based on Win32 platform, so you can easily build it.
The following example is to save logging information into a specified log file.
<Code Example>
You can see at log4cplus.sourceforge.net.
Current version is 1.0.4, released on January 2011.
I tested this library on Visual studio 2005.
It is based on Win32 platform, so you can easily build it.
The following example is to save logging information into a specified log file.
<Code Example>
#include <log4cplus/logger.h>#include <log4cplus/configurator.h>#include <log4cplus/fileappender.h>using namespace log4cplus;int main(){SharedAppenderPtr myAppender(new FileAppender("LogFile.log"));BasicConfigurator config;config.configure();logger = Logger::getInstance("main");logger.addAppender(myAppender);logger.setLogLevel(ALL_LOG_LEVEL);LOG4CPLUS_WARN(logger, "Warning Test");return 0;}
Centos - Sudoers file
In Centos, to use the command "sudo", you add a user information to /etc/sudoers.
For example, Basically root is "root ALL=(ALL) ALL"
You can modify this file for new sudo user.
username ALL=(ALL)ALL
For example, Basically root is "root ALL=(ALL) ALL"
You can modify this file for new sudo user.
username ALL=(ALL)ALL
5/23/2011
Noise Generation
reference: http://homepages.inf.ed.ac.uk/rbf/HIPR2/noise.htm
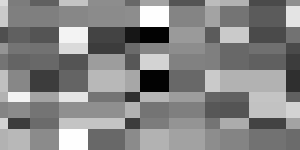
Common Names: Noise Generation
Many image processing packages contain operators to artificially add noise to an image. Deliberately corrupting an image with noise allows us to test the resistance of an image processing operator to noise and assess the performance of various noise filters.

. The impact of the noise on the image is often described by the signal to noise ratio (SNR), which is given by
and
are the variances of the true image and the recorded image, respectively.In many cases, additive noise is evenly distributed over the frequency domain (i.e. white noise), whereas an image contains mostly low frequency information. Hence, the noise is dominant for high frequencies and its effects can be reduced using some kind of lowpass filter. This can be done either with a frequency filter or with a spatial filter. (Often a spatial filter is preferable, as it is computationally less expensive than a frequency filter.)
In the second case of data-dependent noise (e.g. arising when monochromatic radiation is scattered from a surface whose roughness is of the order of a wavelength, causing wave interference which results in image speckle), it is possible to model noise with a multiplicative, or non-linear, model. These models are mathematically more complicated; hence, if possible, the noise is assumed to be data independent.
Detector Noise
One kind of noise which occurs in all recorded images to a certain extent is detector noise. This kind of noise is due to the discrete nature of radiation, i.e. the fact that each imaging system is recording an image by counting photons. Allowing some assumptions (which are valid for many applications) this noise can be modeled with an independent, additive model, where the noise n(i,j) has a zero-mean Gaussian distribution described by its standard deviation (
), or variance. (The 1-D Gaussian distribution has the form shown in Figure 1.) This means that each pixel in the noisy image is the sum of the true pixel value and a random, Gaussian distributed noise value.
Salt and Pepper Noise
Another common form of noise is data drop-out noise (commonly referred to as intensity spikes, speckle or salt and pepper noise). Here, the noise is caused by errors in the data transmission. The corrupted pixels are either set to the maximum value (which looks like snow in the image) or have single bits flipped over. In some cases, single pixels are set alternatively to zero or to the maximum value, giving the image a `salt and pepper' like appearance. Unaffected pixels always remain unchanged. The noise is usually quantified by the percentage of pixels which are corrupted.

Gaussian Noise
We will begin by considering additive noise with a Gaussian distribution. If we add Gaussian noise with
values of 8, we obtain the image
yields
=13 and 20. Compare these images to the original
Gaussian noise can be reduced using a spatial filter. However, it must be kept in mind that when smoothing an image, we reduce not only the noise, but also the fine-scaled image details because they also correspond to blocked high frequencies. The most effective basic spatial filtering techniques for noise removal include: mean filtering, median filtering and Gaussian smoothing. Crimmins Speckle Removal filter can also produce good noise removal. More sophisticated algorithms which utilize statistical properties of the image and/or noise fields exist for noise removal. For example, adaptive smoothing algorithms may be defined which adjust the filter response according to local variations in the statistical properties of the data.
Salt and Pepper Noise
In the following examples, images have been corrupted with various kinds and amounts of drop-out noise. In
For this kind of noise, conventional lowpass filtering, e.g. mean filtering or Gaussian smoothing is relatively unsuccessful because the corrupted pixel value can vary significantly from the original and therefore the mean can be significantly different from the true value. A median filter removes drop-out noise more efficiently and at the same time preserves the edges and small details in the image better. Conservative smoothing can be used to obtain a result which preserves a great deal of high frequency detail, but is only effective at reducing low levels of noise.
A. Jain Fundamentals of Digital Image Processing, Prentice Hall, 1989, pp 244 - 253, 273 - 275.
E. Davies Machine Vision: Theory, Algorithms and Practicalities, Academic Press, 1990, pp 29 - 30, 40 - 47, 493.
B. Horn Robot Vision, MIT Press, 1986, Chap. 2.
A. Marion An Introduction to Image Processing, Chapman and Hall, 1991, Chap. 5.
Noise Generation
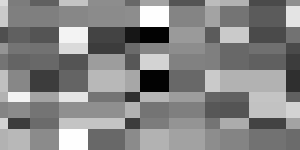
Common Names: Noise Generation
Brief Description
Real world signals usually contain departures from the ideal signal that would be produced by our model of the signal production process. Such departures are referred to as noise. Noise arises as a result of unmodelled or unmodellable processes going on in the production and capture of the real signal. It is not part of the ideal signal and may be caused by a wide range of sources, e.g. variations in the detector sensitivity, environmental variations, the discrete nature of radiation, transmission or quantization errors, etc. It is also possible to treat irrelevant scene details as if they are image noise (e.g. surface reflectance textures). The characteristics of noise depend on its source, as does the operator which best reduces its effects.Many image processing packages contain operators to artificially add noise to an image. Deliberately corrupting an image with noise allows us to test the resistance of an image processing operator to noise and assess the performance of various noise filters.

How It Works
Noise can generally be grouped into two classes:- independent noise.
- noise which is dependent on the image data.
The noise n(i,j) is often zero-mean and described by its variance

where




One kind of noise which occurs in all recorded images to a certain extent is detector noise. This kind of noise is due to the discrete nature of radiation, i.e. the fact that each imaging system is recording an image by counting photons. Allowing some assumptions (which are valid for many applications) this noise can be modeled with an independent, additive model, where the noise n(i,j) has a zero-mean Gaussian distribution described by its standard deviation (

Figure 1 1-D Gaussian distribution with mean 0 and standard deviation 1
Salt and Pepper Noise
Another common form of noise is data drop-out noise (commonly referred to as intensity spikes, speckle or salt and pepper noise). Here, the noise is caused by errors in the data transmission. The corrupted pixels are either set to the maximum value (which looks like snow in the image) or have single bits flipped over. In some cases, single pixels are set alternatively to zero or to the maximum value, giving the image a `salt and pepper' like appearance. Unaffected pixels always remain unchanged. The noise is usually quantified by the percentage of pixels which are corrupted.

Guidelines for Use
In this section we will show some examples of images corrupted with different kinds of noise and give a short overview of which noise reduction operators are most appropriate. A fuller discussion of the effects of the operators is given in the corresponding worksheets.
We will begin by considering additive noise with a Gaussian distribution. If we add Gaussian noise with

Increasing

and
for

Gaussian noise can be reduced using a spatial filter. However, it must be kept in mind that when smoothing an image, we reduce not only the noise, but also the fine-scaled image details because they also correspond to blocked high frequencies. The most effective basic spatial filtering techniques for noise removal include: mean filtering, median filtering and Gaussian smoothing. Crimmins Speckle Removal filter can also produce good noise removal. More sophisticated algorithms which utilize statistical properties of the image and/or noise fields exist for noise removal. For example, adaptive smoothing algorithms may be defined which adjust the filter response according to local variations in the statistical properties of the data.

In the following examples, images have been corrupted with various kinds and amounts of drop-out noise. In
pixels have been set to 0 or 255 with probability p=1%. In
pixel bits were flipped with p=3%, and in
5% of the pixels (whose locations are chosen at random) are set to the maximum value, producing the snowy appearance.
For this kind of noise, conventional lowpass filtering, e.g. mean filtering or Gaussian smoothing is relatively unsuccessful because the corrupted pixel value can vary significantly from the original and therefore the mean can be significantly different from the true value. A median filter removes drop-out noise more efficiently and at the same time preserves the edges and small details in the image better. Conservative smoothing can be used to obtain a result which preserves a great deal of high frequency detail, but is only effective at reducing low levels of noise.
Interactive Experimentation
You can interactively experiment with this operator by clicking here.Exercises
- The image
is a binary chessboard image with 2% of drop-out noise. Which operator yields the best results in removing the noise?
- The image
is the same image corrupted with Gaussian noise with a variance of 180. Is the operator used in Exercise 1 still the most appropriate? Compare the best results obtained from both noisy images.
- Compare the images achieved by median filter and mean filter filtering
with the result that you obtain by applying a frequency lowpass filter to the image. How does the mean filter relate to the frequency filter? Compare the computational costs of mean, median and frequency filtering.
References
R. Gonzales and R. Woods Digital Image Processing, Addison Wesley, 1992, pp 187 - 213.A. Jain Fundamentals of Digital Image Processing, Prentice Hall, 1989, pp 244 - 253, 273 - 275.
E. Davies Machine Vision: Theory, Algorithms and Practicalities, Academic Press, 1990, pp 29 - 30, 40 - 47, 493.
B. Horn Robot Vision, MIT Press, 1986, Chap. 2.
A. Marion An Introduction to Image Processing, Chapman and Hall, 1991, Chap. 5.
Subscribe to:
Posts (Atom)